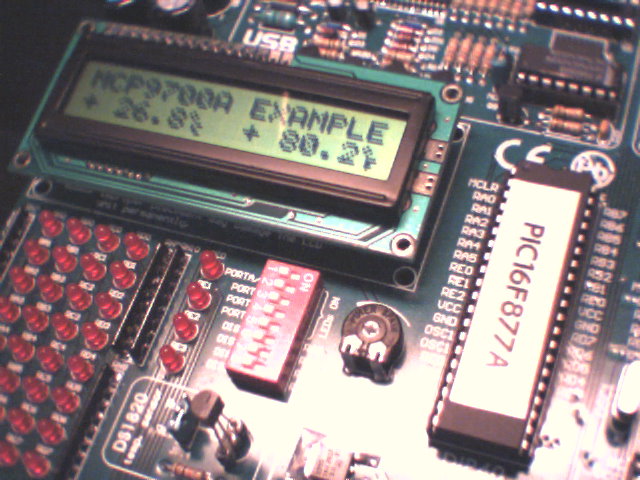
You already know my silicon diode thermometer, this time I'm using a Microchip MCP9700A analog temperature sensor : cheap and easy to use, factory calibrated, it is plug and play.
Let's plug it to an EasyPIC4 to see how we can play with it !
the sensor : Microchip MCP9700A Thermistor
The MCP9700A is a low power linear active thermistor from Microchip, it features :
- a tiny package, only 3 pins : Ground, power supply and output
- a temperature measurement range from -40°C to +125°C with +/- 2°C accuracy
- a power supply range from 2.3V to 5.5V with a very low operating current : 6µA !
- a +10mV/°C temperature coefficient
It is designed to be directly connected to a PIC analog input :
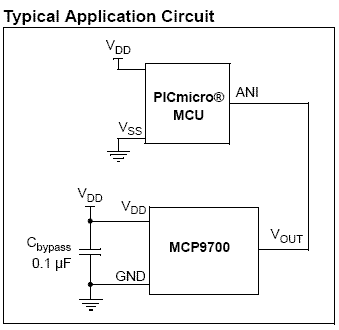 MCP9700A circuit example (from Microchip datasheet)
Its linear output simplifies software : 500mV ouput is 0°C, and 10mV is 1°C :
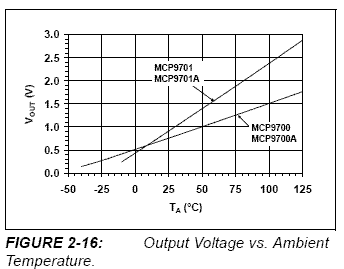 MCP9700A output voltage curve (from Microchip datasheet)
Other similar sensors of the same family are MCP9700, MCP9701, MCP9701A.
To learn more about these sensors, please read the datasheet : http://ww1.microchip.com/downloads/en/DeviceDoc/21942c.pdf
Plug and play
If you have an EasyPIC board, just put the MCP9700A sensor in place of the DS1820 sensor this way :
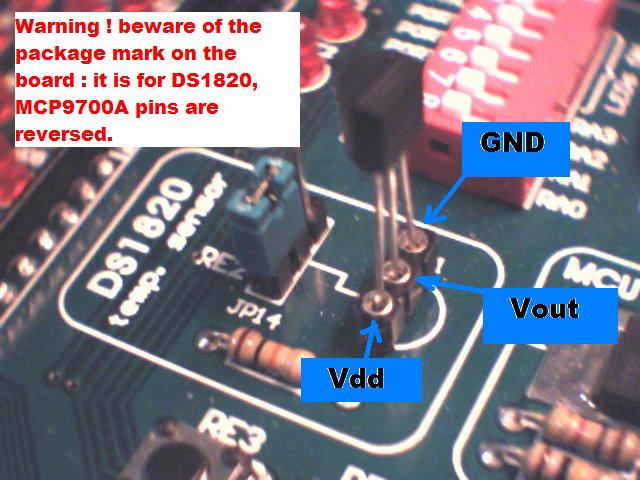
Take care to plug the MCP9700A correctly : the flat side of the package must be on the D1820 curved side !
Then connect JP14 to RE2. That's all !
C source code example
Here is a mikroC source code example to display temperature in Celcius and Fahrenheit degrees on a LCD, using a MCP9700A temperature sensor. If you don't have mikroC compiler you can download it from : http://www.mikroe.com/en/compilers/mikroc/pic/
As you can see, I took advantages of the new mikroC LCD custom character generator to build symbols for °C and °F, as well as new "export code to HTML" feature of mikroC V7.0.0.3 to add color to the source code for better clarity :
#define INT_RANGE 1000 #define DEC_RANGE 10 unsigned int cntr ;
long temp ; int fahr ; int ref = 1024 ; const char characterC[] = {8,20,8,0,3,4,4,3}; const char characterF[] = {8,20,8,0,7,4,6,4}; void CustomChar(const char *def, unsigned char n, char pos_row, char pos_char)
{
char i ;
LCD_Cmd(64 + n * 8) ;
for(i = 0 ; i<=7 ; i++)
{
LCD_Chr_Cp(def[i]) ;
}
LCD_Cmd(LCD_RETURN_HOME) ;
LCD_Chr(pos_row, pos_char, n) ;
}
void LCD_printFix(unsigned int v)
{
unsigned int w ;
unsigned int d ;
unsigned char n ;
unsigned char blk = 1 ; if(v >= 0)
{
LCD_Chr_Cp('+') ;
}
else
{
LCD_Chr_Cp('-') ;
}
v = abs(v) ;
w = v / DEC_RANGE ;
for(d = INT_RANGE / 10 ; d > 0 ; d /= 10)
{
n = (w / d) % 10 ;
if(n)
{
blk = 0 ;
}
if(blk)
{
LCD_Chr_Cp(' ') ;
}
else
{
LCD_Chr_Cp('0' + n) ;
}
}
LCD_Chr_Cp('.') ;
w = v % DEC_RANGE ;
for(d = DEC_RANGE / 10 ; d > 0 ; d /= 10)
{
LCD_Chr_Cp('0' + (w / d) % 10) ;
}
}
void interrupt(void)
{
if(INTCON.T0IF) {
cntr++ ; INTCON.T0IF = 0 ; }
}
void main()
{
ADCON1 = 0x00 ; TRISA = 0xff ; TRISD = 0 ; LCD_Init(&PORTD) ; LCD_Cmd(Lcd_CLEAR) ; LCD_Cmd(Lcd_CURSOR_OFF) ; LCD_Out(1, 1, "MCP9700A EXAMPLE"); OPTION_REG = 0x80 ; INTCON = 0xA0 ; for(;;) {
if(cntr >= 4000) {
temp = Adc_Read(7) * 10 - ref ; temp *= 488 ;
temp /= 1000 ;
fahr = ((9 * temp) / 5 ) + 320 ; LCD_Out(2, 1, "") ;
LCD_printFix(temp) ;
CustomChar(characterC, 0, 2, 7) ;
LCD_Out(2, 10, "") ;
LCD_printFix(fahr) ;
CustomChar(characterF, 1, 2, 16) ;
cntr = 0 ; }
}
}
|
Is it plugged ? Then play !
It should work at first try, this is what you should get on your LCD screen :
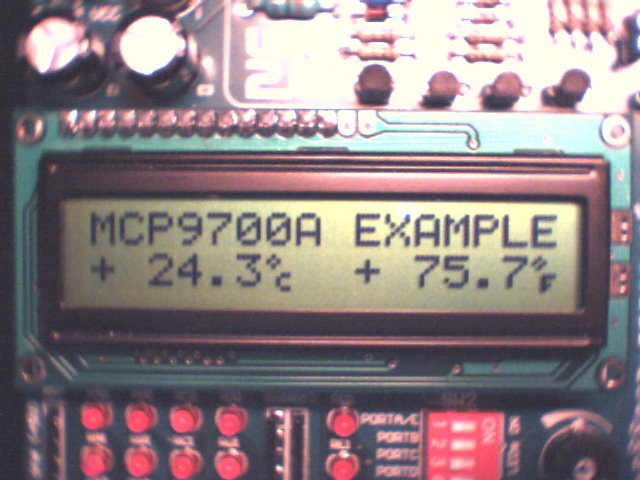
Plug and play, as I said 
This sensor can take place in applications where relative temperature changes are monitored, rather than absolute temperature : computer fan control, home appliance, motors and batteries temperature control for robotics...
Please report bugs, comments or suggestions in my forum.
|